Learning Godot – Pong – Day 1
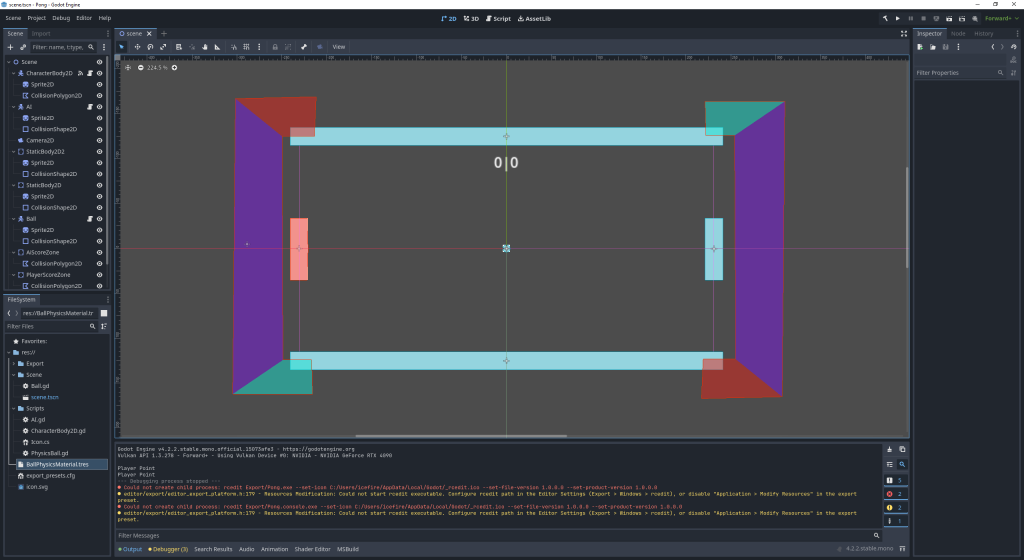
I made Pong in godot. It wasn’t the hardest process. However, I struggled the most trying to understand what methods I could call. I also proud that my pong game works better than the pong example for most gameplay mechanics.
With that said, If I was to re-do this I would want to use Art assets and see if I can give the NPC prediction for bounces as it’s using the first AI code I’ve ever learned. Which is to just follow the target. Also the paddles can move in the X coordinate in the wrong situations. There are a lot of ways I could fix it. But I want to do bigger and better things.
The NPC
extends CharacterBody2D
const SPEED = 300.0
const JUMP_VELOCITY = -400.0
var target_velocity = Vector2.ZERO
var lerp_factor = 0.1 # Adjust this value to control the smoothness
@onready var character_body = get_node("/root/Scene/Ball")
func _ready():
#character_body = get_node("/root/Scene/Ball")
if character_body:
var y_coordinate = character_body.position.y
else:
print("Ball not found.")
func _physics_process(delta):
if not character_body:
print("Ball not found.")
character_body = get_node("/root/Scene/Ball")
var direction_y = character_body.position.y - position.y
var normalized_direction_y = sign(direction_y)
# Calculate the target velocity
target_velocity.y = normalized_direction_y * SPEED
# Interpolate the current velocity towards the target velocity
velocity.y = lerp(velocity.y, target_velocity.y, lerp_factor)
move_and_slide()
The Player
extends CharacterBody2D
const SPEED = 300.0
const JUMP_VELOCITY = -400.0
# Get the gravity from the project settings to be synced with RigidBody nodes.
var gravity = ProjectSettings.get_setting("physics/2d/default_gravity")
func _physics_process(delta):
# Add the gravity.
#if not is_on_floor():
# velocity.y += gravity * delta
# Handle jump.
if Input.is_action_just_pressed("ui_accept") and is_on_floor():
velocity.y = JUMP_VELOCITY
# Get the input direction and handle the movement/deceleration.
# As good practice, you should replace UI actions with custom gameplay actions.
var leftRight = Input.get_axis("ui_left", "ui_right")
var upDown = Input.get_axis("ui_up", "ui_down")
leftRight = 0; #Disable Movement
var direction = Vector2(leftRight, upDown)
if direction.length() > 1:
direction = direction.normalized()
#velocity.x = direction.x * SPEED
#velocity.y = direction.y * SPEED
velocity = direction * SPEED
move_and_slide()
The Ball
extends CharacterBody2D
var centerLocation = Vector2(0,0)
# Speed and direction of the ball
var SPEED = 200
var SPEED_INCREASE = 15
var Bounce = 0
var points = Vector2(0,0)
#var velocity = Vector2(1, 1).normalized()
# Reference to the specific StaticBody2D
@onready var Aigoal_zone = get_node("/root/Scene/AiScoreZone")
@onready var Playergoal_zone = get_node("/root/Scene/PlayerScoreZone")
@onready var Score = get_node("/root/Scene/Score/Label")
# Called when the node enters the scene tree for the first time.
func _ready():
pass # Replace with function body.
position = centerLocation
velocity = Vector2(1, 1).normalized()
# Called every frame. 'delta' is the elapsed time since the previous frame.
func _process(delta):
# Move the ball
var motion = velocity * (SPEED+SPEED_INCREASE*Bounce) * delta
var collision = move_and_collide(motion)
# Check for collision
if collision:
# Reflect the ball's velocity on collision
velocity = velocity.bounce(collision.get_normal())
# Ensure the ball doesn't get stuck by adjusting its position slightly
position += collision.get_normal() * collision.get_remainder()
# Check if the collided object is a CharacterBody2D
if collision.get_collider() is CharacterBody2D:
Bounce+=1
if collision.get_collider() == Aigoal_zone:
print("Player Point")
points.x = points.x + 1
Score.text = str(points.x) + "|" + str(points.y)
_reset()
if collision.get_collider() == Playergoal_zone:
print("Ai Point")
points.y = points.y + 1
Score.text = str(points.x) + "|" + str(points.y)
_reset()
#move_and_slide()
func _reset():
position = centerLocation
Bounce = 0
Download
I’ll attach the exported project below
Download Here
I didn’t have a ton to write about but figured I would show that I’m alive since it’s been a year since my last post.